Docker cheat sheet
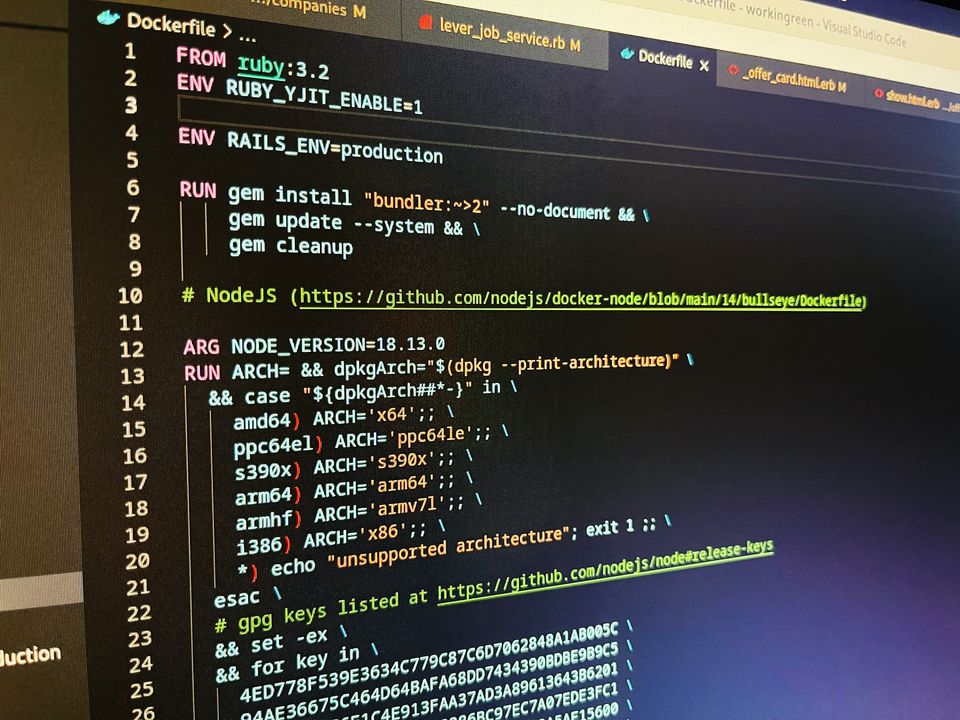
Docker has been out there since 2013. During these past 10 years, it underwent rapid development phases and regular API changes (I remember it quite well from 2014). Even though it's one of the most popular technologies that software developers are using every day, and it runs a lot of software now, many people still can't get out 100% of it.
Because of that, I've decided to create a cheat sheet that you can use to improve your docker skills!
Images
Just a few words on what Docker images are: A Docker image is the set of processes outlined in the Dockerfile. It is helpful to think of these as templates created by the Dockerfiles. They are arranged in layers on top of each other. When you run a container, you're interacting with the top (latest) layer only.
List images
docker images
List all images
docker images -a
Import docker image from tarball
docker import
Build a docker image
docker build
Tag a docker image
docker tag {image_id} repo:tag
Build a docker image with a tag
docker build -t repo:tag
Create an image from a container
docker commit
Remove a single image
docker rmi {image_id}
Load docker image from stdin, including tags
docker import
See an image history
docker history {image_id}
Load a docker image from a file
docker load < archive.tar.gz
Push an image to the registry
docker push repo:tag
Pull an image from the registry
docker pull repo:tag
Remove dangling images
docker image prune
Remove all images that are not used by any container
docker image prune -a
Find an image in the official repository
docker search text
Containers
To simplify, we can say that when a Docker image is instantiated, it becomes a container. By creating an instance that draws on system resources like memory, the container begins to carry out whatever processes are together within the container.
Create a docker container (without starting it)
docker create
Rename a docker container
docker rename
Create and start a docker container
docker run
Create and start a container with a custom name and detach it
docker start --name your-name -d repo:tag
Delete a docker container
docker rm {container_name}
Update the container's limit
docker update
Remove the container once it stops
docker run --rm
Remove the container together with the volumes associated with it
docker rm -v
Start the container
docker start {container_name}
Stop the container
docker stop {container_name}
Restart the container
docker restart {container_name}
Send SIGKILL to the container
docker kill {container_name}
Attach to running container
docker attach {container_name}
List the running containers
docker ps
List all the containers, including stopped
docker ps -a
See docker stats. Provide the container name to show stats for that one only.
docker stats
// docker stats {container_name}
See container logs; add -f to follow logs.
docker logs {container_name}
// docker logs -f {container_name}
Inspect details of container - IP address, volumes attached, ENV variables
docker inspect {container_name}
Check what ports the container listens on
docker port {container_name}
Display running processes in the container
docker top {container-name}
Copy files between a container and a host
docker cp
Execute a command in a container
docker exec
Networks
Docker allows you to create a network that makes connecting to and between containers easier. There are three types of networks in docker - bridge, host, and none. By default, the new container is launched in bridge mode. To establish communication between containers, you should create a network for that.
Create a docker network.
docker network create {name}
List all docker networks.
docker network ls
Remove a docker network with a given name.
docker network rm {name}
Get details about the network (subnet, gateway, scope, type, etc.)
docker network inspect {name}
Connect a container to the network
docker network connect {network} {container}
Disconnect a container from the network
docker network disconnect {network} {container}
Docker compose
For years, if you wanted to run a bunch of docker containers grouped for a project, talk to each other, be dependent on each other, and have the ability to control the entire stack in a simple way, there was a docker-compose project (compose v1), which was a built-in python. Now we have compose v2, rewritten it to Go, and been part of Docker Desktop and as a plugin for Linux. The main difference is a compose
subcommand of docker
instead of being another binary. v2 is backward compatible, so if you're already familiar with docker-compose
you'll be fine.
Run a multi-container application
docker compose up
- you can specify a config file with -f
argument and run it in the background with -d
argument
docker compose -f my-compose.yml up -d
- will use my-compose.yml
file as a definition and set up in the background.
List all running containers.
docker compose ps
Stop an entire set of containers
docker compose stop
Start an entire set of containers again
docker compose start
Restart an entire set of containers
docker compose restart
Stop and remove an entire set of containers
docker compose down
Build containers
docker compose build
- you can use Dockerfile instead of the existing image.
Sample docker-compose file for Rails project:
services:
db:
image: postgres
volumes:
- ./tmp/db:/var/lib/postgresql/data
environment:
POSTGRES_PASSWORD: password
web:
build: .
command: bash -c "rm -f tmp/pids/server.pid && bundle exec rails s -p 3000 -b '0.0.0.0'"
volumes:
- .:/myapp
ports:
- "3000:3000"
depends_on:
- db
The example above will create a multi-container application consisting of two services:
- db service that will use the latest postgres image and mount ./tmp/db
directory as /var/lib/postgresql/data
in the container.
- web service that will use Dockerfile in the current working directory to build a docker image for the Ruby on Rails application. It will expose port 3000 to use on the host system and depends on db service, so db service will be created and run first.
Source of well-defined docker-compose examples: https://github.com/docker/awesome-compose (it's an official repository maintained by docker) - there are examples for popular services like WordPress, ELK stack, or Wireguard server.